Test Settings
The TestSettings
is used to configure how you want Element to behave during a test run, including wait timing, timeouts, browser agent, window size, and much more.
Settings are specified by exporting a const settings
from your test script, and loaded when your test script is first evaluated by the test runner.
Example:
See DEFAULT SETTINGS for a list of the default value for each setting.
#
Setting Properties#
actionDelaynumber
(Optional) Specifies the time (in seconds) to wait between each action call.
Waiting between actions simulates the behaviour of a real user as they read, think and act on the page's content.
#
blockedDomainsstring\[]
(Optional) Blocks requests to a list a domains. Accepts partial matches using *
or any matcher accepted by Micromatch.
Matching is applied to the hostname
only, unless the blocked domain contains a :
in which case it will match against hostname
and port
.
Example: ["*.google-analytics.com", "*:1337"]
#
browserSince Element 2.0, you can set the browser type used to run the test, either using one of the 3 browser types bundled with Playwright, including 'chromium'
, 'firefox'
and 'webkit'
or using 'chrome'
installed on the machine. Defaults to 'chromium'
.
Note that using 'chrome'
only works if Google Chrome is installed in the default path:
- On Windows: C:/Program Files (x86)/GoogleChrome/Applicationchrome.exe
- On MacOS: /Applications/Google Chrome.app/Contents/MacOS/Google Chrome
- On Flood Grid: /usr/bin/google-chrome-stable
Otherwise, you need to specify the custom path with executablePath property in browserLaunchOptions setting.
#
browserLaunchOptionsObject
(Optional) Sets additional launch options on the browser. This will allow you to specify a custom Chromium-based browser type used to run the test, like Microsoft Edge or Brave, rather than the 3 bundled ones.
Properties
- executablePath
string
path to the installation folder of a custom browser based on Chromium. If set, Element will ignore the browser settings, and use this custom browser instead. - downloadsPath
string
if specified, accepted downloads are downloaded into this directory. Otherwise, temporary directory is created and is deleted when browser is closed. - firefoxUserPrefs
Object
Firefox user preferences. Learn more about the Firefox user preferences here - proxy
Object
Network proxy settings.- server
string
Proxy to be used for all requests. HTTP and SOCKS proxies are supported, for examplehttp://myproxy.com:3128
orsocks5://myproxy.com:3128
. Short formmyproxy.com:3128
is considered an HTTP proxy. - bypass
string
Optional coma-separated domains to bypass proxy, for example ".com, chromium.org, .domain.com". - username
string
Optional username to use if HTTP proxy requires authentication. - password
string
Optional password to use if HTTP proxy requires authentication.
- server
chromeVersion (DEPRECATED since version 2.0)#
puppeteer
or stable
(Optional) Specifies a version of Google Chrome to run the test
This setting is no longer supported since version 2.0. Instead, you can use browser setting or executablePath property in browserLaunchOptions setting to specify the target browser.
#
clearCachefalse
| true
(Optional) Specifies whether Browser cache should be cleared after each test loop.
#
clearCookiesfalse
| true
(Optional) Specifies whether cookies should be cleared after each test loop.
#
consoleFilterConsoleMethod(Optional) Specify which console methods to filter out. By default no console methods are filtered.
This setting can be useful for very noisy tests. When a method is filtered, it still works as normal but the message will be omitted from the Element output.
#
descriptionstring
(Optional) Speicifies the description of the test specified in the comments section
#
devicestring
(Optional) Specifies a device to emulate with browser device emulation.
#
disableCachefalse
| true
(Optional) Disables browser request cache for all requests.
#
durationnumber
(Optional) Maximum duration to run the test for.
Note that when running a load test via Flood, the duration of the load test takes precedence over this setting.
Defaults to -1
for no timeout.
#
extraHTTPHeadersObject
(Optional) Specifies a set of extra HTTP headers to set before each test loop.
If this setting is undefined, the extra HTTP headers are left as-is between iterations.
#
ignoreHTTPSErrorsfalse
| true
(Optional) Whether to ignore HTTPS errors during navigation. Defaults to false
#
incognitofalse
| true
(Optional) Controls whether each iteration should run within an Incognito window instead of a normal
window. The Incognito session will be destroyed between each loop.
#
launchArgsstring[]
Additional arguments to pass to the browser instance.
The list of Chromium flags can be found here
#
loopCountnumber
(Optional) Number of times to run this test.
Defaults to -1
(or Infinity
) for an unlimited number of loops.
#
namestring
(Optional) Speicifies the name of the test specified in the comments section
#
responseTimeMeasurementResponseTiming (Optional) Configures how we record response time for each step.
Possible values:
"page"
: Record the document loading response time. This is usually what you consider response time on paged web apps."network"
: Takes the mean response time of all network requests which occur during a step. This is useful for Single Page Application which don't actually trigger a navigation."step"
: (Default) Records the wall clock time of a step. This is useful for Single Page Application which don't actually trigger a navigation."stepWithThinkTime"
: Records the wall clock time of a step includingactionDelay
time.
#
screenshotOnFailurefalse
| true
(Optional) Take a screenshot of the page when a command fails, to aid in debugging.
Screenshots are saved to /flood/result/screenshots
in the test archive.
#
stages Array
(Optional) Since Element 2.0, you can specify the target number of Virtual Users (VUs), each running in a separate browser instance, to ramp up or down to for a specific period. This would be helpful for a local load test at a small scale.
Note that this setting is only applicable when running test scripts locally with the option --mu
.
For example, the below settings would have Element ramp up from 1 to 5 VUs (browser instances) for 3 minutes, then stay flat at 5 VUs for 5 minutes, then continue to ramp up from 5 to 10 VUs over the next 10 minutes before finally ramp down to 3 VUs for another 3 minutes.
When running with multiple users locally, you will get a table which reports the load-testing metrics after the test finishes.
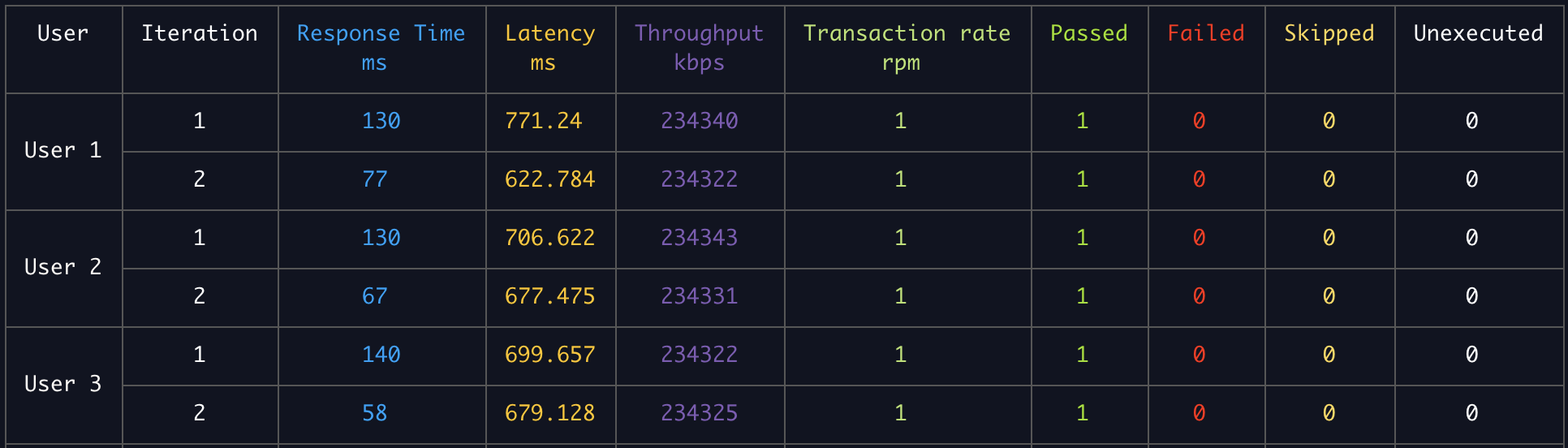
#
stepDelay number
(Optional) Specifies the time (in seconds) to wait after each step.
#
userAgentstring
(Optional) Specifies a custom User Agent (UA) string to send.
#
viewportObject
Set the viewport with the below properties:
- width
number
page width in pixels. Required. - height
number
page height in pixels. Required. - deviceScaleFactor
number
Specify device scale factor (can be thought of as dpr). Defaults to 1. - isMobile
boolean
Whether the meta viewport tag is taken into account. Defaults tofalse
. - hasTouch
boolean
Specifies if viewport supports touch events. Defaults tofalse
. - isLandscape
boolean
Specifies if viewport is in landscape mode. Defaults tofalse
.
#
waitTimeoutnumber
(Optional) Global wait timeout applied to all wait tasks.
#
waitUntil : (Optional)- present: only checks for presence in the DOM
- visible: waits until the element is visible on the page and is painted
- ready: waits until the element is painted and not disabled
#
Console MethodSpecifies a console
method
#
ResponseTimingSpecifies a method for recording response times.
literal | description |
---|---|
step | (Default) Records the wall clock time of a step. This is useful for Single Page Application which don't actually trigger a navigation. |
page | Record the document loading response time. This is usually what you consider response time on paged web apps. |
network | (Experimental) Takes the mean response time of all network requests which occur during a step. This is useful for Single Page Application which don't actually trigger a navigation. |
stepWithThinkTime | "stepWithThinkTime" : Records the wall clock time of a step including actionDelay time. |
#
setup(settings)settings
<[TestSettings]>returns: <void>
Declares the settings for the test, overriding the settings constant exported in the test script.
This is a secondary syntax for export const settings = {}
which functions exactly the same way.
Example:
#
DEFAULT SETTINGSThe default settings for a Test. Any settings you provide are merged into these defaults.
Name | Default Value | Comment |
---|---|---|
actionDelay | '2s' | |
blockedDomains | [] | |
browser | 'chromium' | Since version 2.0 |
browserLaunchOptions | {} | Since version 2.0 |
chromeVersion | DEPRECATED since version 2.0 | |
clearCache | false | |
clearCookies | true | |
consoleFilter | [] | By default, don't filter any console messages from the browser |
description | '' | |
device | null | |
disableCache | false | |
duration | -1 | Defaults to -1 for unlimited number of loops |
extraHTTPHeaders | {} | |
ignoreHTTPSErrors | false | |
incognito | false | |
launchArgs | [] | |
loopCount | Infinity | Equivalent to -1 |
name | 'Element Test' | |
responseTimeMeasurement | 'step' | |
screenshotOnFailure | true | |
stages | [] | Since version 2.0 |
stepDelay | '6s' | |
userAgent | '' | |
waitTimeout | '30s' | |
waitUntil | false |